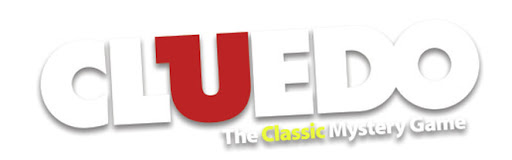 |
RCL - RoboCLuedo
v1.0
Francesco Ganci - S4143910 - Experimental Robotics Lab - Assignment 1
|
Go to the documentation of this file.
68 #include "std_srvs/Trigger.h"
69 #include "armor_msgs/ArmorDirective.h"
70 #include "armor_msgs/ArmorDirectiveList.h"
71 #include "armor_msgs/ArmorDirectiveReq.h"
72 #include "armor_msgs/ArmorDirectiveRes.h"
73 #include "armor_msgs/QueryItem.h"
74 #include "robocluedo_msgs/AddHint.h"
75 #include "robocluedo_msgs/Hypothesis.h"
76 #include "robocluedo_msgs/FindConsistentHypotheses.h"
77 #include "robocluedo_msgs/DiscardHypothesis.h"
84 #define SERVICE_INTERFACE_ADD_HINT "/cluedo_armor/add_hint"
85 #define SERVICE_INTERFACE_FIND_CONSISTENT_HYP "/cluedo_armor/find_consistent_h"
86 #define SERVICE_INTERFACE_WRONG_HYPOTHESIS "/cluedo_armor/wrong_hypothesis"
87 #define SERVICE_INTERFACE_SAVE_ONTOLOGY "/cluedo_armor/backup"
89 #define ONTOLOGY_PARAM "cluedo_path_owlfile"
90 #define PARAM_ONTOLOGY_BACKUP_PATH "cluedo_path_owlfile_backup"
91 #define OUTLABEL "[cluedo_armor_interface]"
92 #define OUTLOG( msg ) ROS_INFO_STREAM( OUTLABEL << " " << msg );
93 #define LOGSQUARE( str ) "[" << str << "] "
94 #define SS( this_string ) std::string( this_string )
95 #define SSS( this_thing ) std::to_string( this_thing )
105 bool fileExist( std::string path )
107 return (std::ifstream(path)).good();
127 std::vector<std::string> to_return;
129 for(
auto it1 = list1.begin(); it1 != list1.end(); ++it1 )
132 for(
auto it2 = list2.begin(); it2 != list2.end(); ++it2 )
142 to_return.push_back( *it1 );
174 bool ServiceAddHint( robocluedo_msgs::AddHint::Request&
hint, robocluedo_msgs::AddHint::Response& success )
179 std::string hypname =
"";
182 armor->
AddIndiv( hypname,
"HYPOTHESIS",
false );
187 if(
hint.property ==
"where" )
189 else if(
hint.property ==
"who" )
191 else if(
hint.property ==
"what" )
195 OUTLOG(
"ERROR: not a valid hint property." );
196 success.success =
false;
203 OUTLOG(
"set object property " <<
"(" << hypname <<
", " <<
hint.Belem <<
"):" <<
hint.property );
209 success.success =
true;
236 std::vector<std::string> list_to_return;
249 hyplist.hyp = std::vector<robocluedo_msgs::Hypothesis>( );
250 robocluedo_msgs::Hypothesis h;
251 for( std::string hptag : list_to_return )
258 hyplist.hyp.push_back( h );
279 bool DiscardHypothesis( robocluedo_msgs::DiscardHypothesis::Request& tag, robocluedo_msgs::DiscardHypothesis::Response& success )
312 success.success =
false;
315 OUTLOG(
"ERROR: " << success.message );
319 std::string save_path;
322 success.success =
true;
324 OUTLOG(
"Ontology save here -> " << save_path );
340 int main(
int argc,
char* argv[] )
342 ros::init( argc, argv,
"cluedo_armor_interface" );
346 std::string ontology_file_path;
353 if( !fileExist( ontology_file_path ) )
361 OUTLOG(
"loading armor ..." );
365 OUTLOG(
"ERROR: unable to load aRMOR!" );
368 armor = &armorcluedo;
#define SERVICE_INTERFACE_WRONG_HYPOTHESIS
bool AddIndiv(std::string indivname, std::string classname, bool makeDisjoint=true)
add an individual to the ontology
#define SERVICE_INTERFACE_FIND_CONSISTENT_HYP
additional aRMOR C++ interface
bool SetObjectProperty(std::string prop, std::string Aelem, std::string Belem)
set a property true
#define SERVICE_INTERFACE_SAVE_ONTOLOGY
bool Init(std::string ontologyPath)
initizalize the interface
std::vector< std::string > GetValuedOfIndiv(std::string prop, std::string indivname)
get the values of a property related to a gven individual
#define PARAM_ONTOLOGY_BACKUP_PATH
bool ServiceBackupOntology(std_srvs::Trigger::Request &emptyrequest, std_srvs::Trigger::Response &success)
implementation of service SERVICE_INTERFACE_SAVE_ONTOLOGY
bool ServiceAddHint(robocluedo_msgs::AddHint::Request &hint, robocluedo_msgs::AddHint::Response &success)
implementation of service SERVICE_INTERFACE_ADD_HINT
bool TestInterface()
check the status of the interface
bool UpdateOntology()
send the command REASON
std::vector< std::string > FindInconsistentHypotheses()
find all the inconsistent hypotheses
int main(int argc, char *argv[])
ROS node main.
additional utilities for aRMOR
bool DiscardHypothesis(robocluedo_msgs::DiscardHypothesis::Request &tag, robocluedo_msgs::DiscardHypothesis::Response &success)
implementation of service SERVICE_INTERFACE_WRONG_HYPOTHESIS
hint
the message from the oracle, if any
bool SaveOntology(std::string path)
save the ontology on file
bool ServiceFindConsistentHypotheses(robocluedo_msgs::FindConsistentHypotheses::Request &empty, robocluedo_msgs::FindConsistentHypotheses::Response &hyplist)
implementation of service SERVICE_INTERFACE_FIND_CONSISTENT_HYP
bool ExistsIndiv(std::string indivname)
check if an individual exists
A minimal C++ client for aRMOR.
#define SERVICE_INTERFACE_ADD_HINT
bool RemoveHypothesis(std::string hypTag)
discard one hypothesis
std::vector< std::string > FindCompleteHypotheses()
find all the complete hypotheses
std::vector< std::string > PerformDifferenceBetween(std::vector< std::string > list1, std::vector< std::string > list2)
delete all the occurrences of list2 inside list1