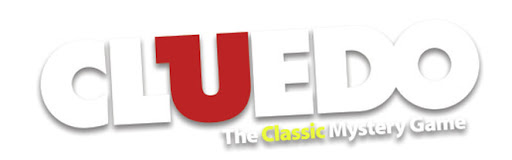 |
RCL - RoboCLuedo
v1.0
Francesco Ganci - S4143910 - Experimental Robotics Lab - Assignment 1
|
Go to the documentation of this file.
14 #ifndef __H_ARMOR_CLUEDO_H__
15 #define __H_ARMOR_CLUEDO_H__
99 bool Init( std::string ontologyPath );
144 bool AddIndiv( std::string indivname, std::string classname,
bool makeDisjoint =
true );
164 std::vector<std::string>
GetClassOfIndiv( std::string indivname,
bool deep );
215 bool SetObjectProperty( std::string prop, std::string Aelem, std::string Belem );
231 std::vector<std::string>
GetValuedOfIndiv( std::string prop, std::string indivname );
322 std::vector<std::string>
FilterVector( std::vector<std::string>& itemlist );
326 std::vector<std::string> individuals;
329 std::vector<std::string> DiscardHypotheses;
332 bool ExistsItem( std::string item,
const std::vector<std::string>& container );
335 bool TrackIndiv( std::string indivname );
338 void DisjointAllIndiv( std::string from );
342 std::vector<std::string>::iterator GetPositionOf( std::string tag, std::vector<std::string>& list );
bool AddIndiv(std::string indivname, std::string classname, bool makeDisjoint=true)
add an individual to the ontology
bool SetObjectProperty(std::string prop, std::string Aelem, std::string Belem)
set a property true
bool Init(std::string ontologyPath)
initizalize the interface
std::string FilterValue(std::string raw)
rewrite a string like '<uri#value>' into 'value'
std::vector< std::string > GetValuedOfIndiv(std::string prop, std::string indivname)
get the values of a property related to a gven individual
std::vector< std::string > GetIndivOfClass(std::string classname)
find the individuals belonging to a class
~ArmorCluedo()
class destructor
std::vector< std::string > FilterVector(std::vector< std::string > &itemlist)
filter all the strings inside the array
std::vector< std::string > FindInconsistentHypotheses()
find all the inconsistent hypotheses
A minimal C++ client for aRMOR.
ArmorCluedo(bool debugmode=ARMOR_DEFAULT_DEBUGMODE)
class constructor of ArmorCluedo
std::vector< std::string > GetClassOfIndiv(std::string indivname, bool deep)
get the class of a given individual
additional utilities for aRMOR
bool ExistsIndiv(std::string indivname)
check if an individual exists
A minimal C++ client for aRMOR.
bool RemoveHypothesis(std::string hypTag)
discard one hypothesis
std::vector< std::string > FindCompleteHypotheses()
find all the complete hypotheses
#define ARMOR_DEFAULT_DEBUGMODE