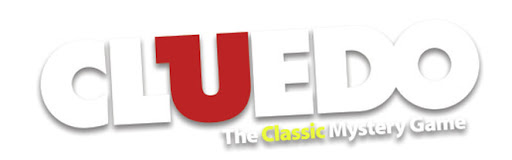 |
RCL - RoboCLuedo
v1.0
Francesco Ganci - S4143910 - Experimental Robotics Lab - Assignment 1
|
Go to the documentation of this file.
22 #include "armor_msgs/ArmorDirective.h"
23 #include "armor_msgs/ArmorDirectiveList.h"
24 #include "armor_msgs/ArmorDirectiveReq.h"
25 #include "armor_msgs/ArmorDirectiveRes.h"
26 #include "armor_msgs/QueryItem.h"
33 #define ONTOLOGY_PARAM "cluedo_path_owlfile"
34 #define SERVICE_ARMOR_SINGLE_REQUEST "/armor_interface_srv"
35 #define SERVICE_ARMOR_MULTIPLE_REQUESTS "/armor_interface_serialized_srv"
36 #define ARMOR_CLIENT_NAME "robocluedo"
37 #define ARMOR_REFERENCE_NAME "cluedo_ontology"
38 #define OUTLABEL "[test_armor_tools] "
44 return (std::ifstream(path)).good();
49 void printList( std::vector<std::string> list, std::string intro =
"" )
52 ROS_INFO_STREAM(
OUTLABEL << intro );
54 if( list.size() == 0 )
56 ROS_INFO_STREAM(
OUTLABEL <<
"size==0" );
61 for(
auto it = list.begin(); it != list.end( ); ++it )
63 ROS_INFO_STREAM(
OUTLABEL <<
"\t" <<
"numero " << idx++ <<
" -> " << *it );
144 armor.
SendCommand(
"DISJOINT",
"CLASS",
"",
"PERSON",
"WEAPON" );
145 armor.
SendCommand(
"DISJOINT",
"CLASS",
"",
"WEAPON",
"PLACE" );
146 armor.
SendCommand(
"DISJOINT",
"CLASS",
"",
"PERSON",
"PLACE" );
149 armor.
SendCommand(
"ADD",
"IND",
"CLASS",
"Jim",
"PERSON" );
150 armor.
SendCommand(
"ADD",
"OBJECTPROP",
"IND",
"who",
"HP3",
"Jim" );
151 armor.
SendCommand(
"ADD",
"IND",
"CLASS",
"Gun",
"WEAPON" );
152 armor.
SendCommand(
"ADD",
"OBJECTPROP",
"IND",
"what",
"HP3",
"Gun" );
153 armor.
SendCommand(
"ADD",
"IND",
"CLASS",
"Stadium",
"PLACE" );
154 armor.
SendCommand(
"ADD",
"OBJECTPROP",
"IND",
"where",
"HP3",
"Stadium" );
156 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"COMPLETED" );
157 ROS_INFO_STREAM(
OUTLABEL <<
"(1) completed hypotheses:" );
159 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"INCONSISTENT" );
160 ROS_INFO_STREAM(
OUTLABEL <<
"(1) inconsistent hypotheses:" );
164 armor.
SendCommand(
"ADD",
"IND",
"CLASS",
"Lounge",
"PLACE" );
166 armor.
SendCommand(
"ADD",
"IND",
"CLASS",
"Study",
"PLACE" );
171 armor.
SendCommand(
"ADD",
"OBJECTPROP",
"IND",
"where",
"HP3",
"Study" );
173 ROS_INFO_STREAM(
OUTLABEL <<
"(2) completed hypotheses:" );
174 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"COMPLETED" );
176 ROS_INFO_STREAM(
OUTLABEL <<
"(2) inconsistent hypotheses:" );
177 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"INCONSISTENT" );
182 armor.
SendCommand(
"DISJOINT",
"IND",
"",
"Lounge",
"HP3" );
184 ROS_INFO_STREAM(
OUTLABEL <<
"(3) un disjoint strano:" );
186 ROS_INFO_STREAM(
OUTLABEL <<
"(3) inconsistent hypotheses:" );
187 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"INCONSISTENT" );
190 ROS_INFO_STREAM(
OUTLABEL <<
"query elementi della classe PLACE" );
191 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"PLACE" );
193 ROS_INFO_STREAM(
OUTLABEL <<
"query proprieta' di hp3" );
194 armor.
SendCommand(
"QUERY",
"IND",
"OBJECTPROP",
"HP3" );
196 ROS_INFO_STREAM(
OUTLABEL <<
"query di tutte le ipotesi" );
197 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"HYPOTHESIS" );
199 ROS_INFO_STREAM(
OUTLABEL <<
"query tutte le classi a cui HP3 apartiene" );
200 armor.
SendCommand(
"QUERY",
"CLASS",
"IND",
"HP3",
"false" );
202 ROS_INFO_STREAM(
OUTLABEL <<
"query solo la classe piu' profonda" );
203 armor.
SendCommand(
"QUERY",
"CLASS",
"IND",
"HP3",
"true" );
206 ROS_INFO_STREAM(
OUTLABEL <<
"salvataggio" );
207 armor.
SendCommand(
"SAVE",
"INFERENCE",
"",
"/root/Desktop/ROBOCLUEDO_ONTOLOGY.owl" );
215 armor.
Init( ontology_file_path );
217 armor.
SendCommand(
"DISJOINT",
"CLASS",
"",
"PERSON",
"WEAPON" );
218 armor.
SendCommand(
"DISJOINT",
"CLASS",
"",
"WEAPON",
"PLACE" );
219 armor.
SendCommand(
"DISJOINT",
"CLASS",
"",
"PERSON",
"PLACE" );
222 ROS_INFO_STREAM(
OUTLABEL <<
"\n\n\n TEST COMANDI DIRETTI ARMOR \n\n\n" );
227 armor.
AddIndiv(
"HP3",
"HYPOTHESIS" );
233 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"COMPLETED" );
234 ROS_INFO_STREAM(
OUTLABEL <<
"(0) completed hypotheses: (dovrebbe essere vuoto)" );
236 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"INCONSISTENT" );
237 ROS_INFO_STREAM(
OUTLABEL <<
"(0) inconsistent hypotheses: (dovrebbe essere vuoto)" );
241 armor.
AddIndiv(
"Stadium",
"PLACE" );
245 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"COMPLETED" );
246 ROS_INFO_STREAM(
OUTLABEL <<
"(1) completed hypotheses: (dovrebbe comparire un'ipotesi)" );
248 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"INCONSISTENT" );
249 ROS_INFO_STREAM(
OUTLABEL <<
"(1) inconsistent hypotheses: (la lista dovrebbe essere vuota)" );
253 armor.
AddIndiv(
"Lounge",
"PLACE" );
258 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"COMPLETED" );
259 ROS_INFO_STREAM(
OUTLABEL <<
"(2) completed hypotheses: (sempre una sola ipotesi)" );
261 armor.
SendCommand(
"QUERY",
"IND",
"CLASS",
"INCONSISTENT" );
262 ROS_INFO_STREAM(
OUTLABEL <<
"(2) inconsistent hypotheses: (dovrebbe spuntare un'ipotesi inconsistente)" );
267 ROS_INFO_STREAM(
OUTLABEL <<
"proprieta' where dell'ipotesi HP3:" );
273 ROS_INFO_STREAM(
OUTLABEL <<
"test esistenza di HP3:" );
274 armor.
SendCommand(
"QUERY",
"IND",
"",
"HP3",
"",
"",
"",
"",
true );
275 ROS_INFO_STREAM(
OUTLABEL <<
"esiste HP3? (atteso: yes) -> " << ( armor.
ExistsIndiv(
"HP3" ) ?
"yes" :
"no" ) );
280 ROS_INFO_STREAM(
OUTLABEL <<
"\n\n\n TEST COMPLETO INTERFACCIA \n\n\n" );
282 ROS_INFO_STREAM(
OUTLABEL <<
"\n\n\t======\tTEST GetClassOfIndiv()" );
283 ROS_INFO_STREAM(
OUTLABEL <<
"classe dell'individual " <<
"Stadium" <<
"(con la maiuscola, scritto correttamente, no deep search, atteso un solo elemento)" );
285 ROS_INFO_STREAM(
"" );
286 ROS_INFO_STREAM(
OUTLABEL <<
"classe dell'individual " <<
"Stadium" <<
"(con la maiuscola, scritto correttamente, usando la deep search, atteso un solo elemento)" );
288 ROS_INFO_STREAM(
"" );
289 ROS_INFO_STREAM(
OUTLABEL <<
"classe dell'individual " <<
"stadium" <<
"(senza la maiuscola, sbagliato, no deep search, atteso 0)" );
291 ROS_INFO_STREAM(
"" );
295 ROS_INFO_STREAM(
OUTLABEL <<
"\n\n\t======\tTEST ExistsIndiv()" );
296 ROS_INFO_STREAM(
OUTLABEL <<
"esiste '" <<
"stadium" <<
"' senza la maiuscola? -> " << ( armor.
ExistsIndiv(
"stadium" ) ?
"yes" :
"no" ) );
297 ROS_INFO_STREAM(
OUTLABEL <<
"esiste '" <<
"Stadium" <<
"' con la maiuscola? -> " << ( armor.
ExistsIndiv(
"Stadium" ) ?
"yes" :
"no" ) );
298 ROS_INFO_STREAM(
OUTLABEL <<
"esiste '" <<
"HP0" <<
"' ? -> " << ( armor.
ExistsIndiv(
"HP0" ) ?
"yes" :
"no" ) );
302 ROS_INFO_STREAM(
OUTLABEL <<
"\n\n\t======\tTEST GetIndivOfClass()" );
303 ROS_INFO_STREAM(
OUTLABEL <<
"tutti gli individuals della classe HYPOTHESIS" <<
"(attesa ... un bel po' di roba)" );
305 ROS_INFO_STREAM(
"" );
306 ROS_INFO_STREAM(
OUTLABEL <<
"tutti gli individuals della classe PLACE" <<
"(attesi 3 elementi)" );
308 ROS_INFO_STREAM(
"" );
311 ROS_INFO_STREAM(
OUTLABEL <<
"\n\n\t======\tTEST GetValuedOfIndiv()" );
312 ROS_INFO_STREAM(
OUTLABEL <<
"proprietà where dell'ipotesi HP3" <<
"(attesi due elementi)" );
314 ROS_INFO_STREAM(
"" );
318 ROS_INFO_STREAM(
OUTLABEL <<
"\n\n\t======\tTEST RemoveHypothesis()" );
319 ROS_WARN_STREAM(
OUTLABEL <<
"NOTA BENE: il comando REMOVE di aRMOR è buggato, non funziona. Per farlo funzionare occorre trovare un workaround nell'interfaccia" );
321 ROS_INFO_STREAM(
OUTLABEL <<
"aggiungo l'ipotesi HPtoremove (uso qui solo comandi dell'interfaccia, non i diretti di aRMOR)" );
322 armor.
AddIndiv(
"HPtoremove",
"HYPOTHESIS",
false );
324 ROS_INFO_STREAM(
OUTLABEL <<
"esiste '" <<
"HPtoremove" <<
"' ? -> " << ( armor.
ExistsIndiv(
"HPtoremove" ) ?
"yes" :
"no" ) );
325 ROS_INFO_STREAM(
OUTLABEL <<
"classe dell'individual " <<
"HPtoremove" <<
"? (atteso un solo elemento)" );
328 ROS_INFO_STREAM(
OUTLABEL <<
"rimozione" );
332 ROS_INFO_STREAM(
OUTLABEL <<
"RemoveHypothesis( ) = " << ( armor.
RemoveHypothesis(
"HPtoremove" ) ?
"yes" :
"no" ) );
334 ROS_INFO_STREAM(
OUTLABEL <<
"esiste ancora '" <<
"HPtoremove" <<
"' ? -> " << ( armor.
ExistsIndiv(
"HPtoremove" ) ?
"yes" :
"no" ) );
338 ROS_INFO_STREAM(
OUTLABEL <<
"\n\n\t======\tTEST FindCompleteHypotheses()" );
343 ROS_INFO_STREAM(
OUTLABEL <<
"\n\n\t======\tTEST FindInconsistentHypotheses()" );
348 std::string save_path;
349 ros::param::get(
"cluedo_path_owlfile_backup", save_path );
357 int main(
int argc,
char* argv[] )
359 ros::init( argc, argv,
"test_armor_tools" );
363 std::string ontology_file_path;
375 ROS_INFO_STREAM(
OUTLABEL <<
"Ontology found! " <<
LOGSQUARE( ontology_file_path ) );
380 ROS_INFO_STREAM(
OUTLABEL <<
"THAT'S ALL FOLKS!" );
void PrintLastRes()
print the last response
bool AddIndiv(std::string indivname, std::string classname, bool makeDisjoint=true)
add an individual to the ontology
additional aRMOR C++ interface
bool SetObjectProperty(std::string prop, std::string Aelem, std::string Belem)
set a property true
bool Init(std::string ontologyPath)
initizalize the interface
std::vector< std::string > GetValuedOfIndiv(std::string prop, std::string indivname)
get the values of a property related to a gven individual
void armorTestSession2(std::string ontology_file_path)
std::vector< std::string > GetIndivOfClass(std::string classname)
find the individuals belonging to a class
bool UpdateOntology()
send the command REASON
bool SendCommand(std::string command, std::string first_spec="", std::string second_spec="", std::string arg1="", std::string arg2="", std::string arg3="", std::string arg4="", std::string arg5="", bool printRequest=false)
fill in a command and send it to aRMOR
A minimal C++ client for aRMOR.
int main(int argc, char *argv[])
std::vector< std::string > GetClassOfIndiv(std::string indivname, bool deep)
get the class of a given individual
void PrintLastReq()
print the last request
bool fileExist(std::string path)
additional utilities for aRMOR
bool SaveOntology(std::string path)
save the ontology on file
bool ExistsIndiv(std::string indivname)
check if an individual exists
void printList(std::vector< std::string > list, std::string intro="")
A minimal C++ client for aRMOR.
void armorTestSession(ArmorTools &armor)
bool RemoveHypothesis(std::string hypTag)
discard one hypothesis
std::vector< std::string > FindCompleteHypotheses()
find all the complete hypotheses