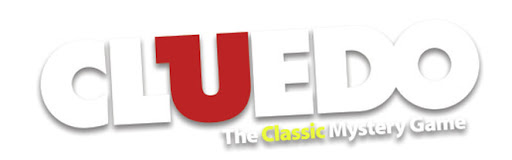 |
RCL - RoboCLuedo
v1.0
Francesco Ganci - S4143910 - Experimental Robotics Lab - Assignment 1
|
Go to the documentation of this file.
14 #ifndef __H_ARMOR_TOOLS_H__
15 #define __H_ARMOR_TOOLS_H__
18 #include "armor_msgs/QueryItem.h"
19 #include "armor_msgs/ArmorDirective.h"
20 #include "armor_msgs/ArmorDirectiveReq.h"
21 #include "armor_msgs/ArmorDirectiveRes.h"
29 #define ARMOR_DEFAULT_URI "http://www.emarolab.it/cluedo-ontology"
30 #define ARMOR_DEFAULT_REASONER "PELLET"
31 #define ARMOR_DEFAULT_CLIENT "armor_client"
32 #define ARMOR_DEFAULT_REFERENCE "cluedo"
33 #define ARMOR_DEFAULT_TIMEOUT 5.00
34 #define ARMOR_DEFAULT_DEBUGMODE true
36 #define ARMOR_SERVICE_SINGLE_REQUEST "/armor_interface_srv"
37 #define ARMOR_SERVICE_MULTIPLE_REQUESTS "/armor_interface_serialized_srv"
39 #define ARMOR_CLASS_LABEL "[armor_tools]"
40 #define ARMOR_INFO( msg ) if( this->DebugMode ) ROS_INFO_STREAM( ARMOR_CLASS_LABEL << " " << msg )
41 #define ARMOR_ERR( msg ) if( this->DebugMode ) ROS_WARN_STREAM( ARMOR_CLASS_LABEL << " ERROR: " << msg )
42 #define ARMOR_CHECK_INTERFACE( returnval ) if( !IsLoadedInterface || !ArmorSrv.exists( ) ) { ARMOR_ERR( "bad interface!" ); return returnval; }
43 #define ARMOR_RES( msg ) msg.response.armor_response
44 #define ARMOR_RES_QUERY( msg ) msg.response.armor_response.queried_objects
46 #define SS( this_string ) std::string( this_string )
47 #define SSS( this_thing ) std::to_string( this_thing )
48 #define BOOL_TO_CSTR( booleanvalue ) ( booleanvalue ? "true" : "false" )
49 #define LOGSQUARE( str ) "[" << str << "] "
150 std::string first_spec =
"",
151 std::string second_spec =
"",
152 std::string arg1 =
"",
153 std::string arg2 =
"",
154 std::string arg3 =
"",
155 std::string arg4 =
"",
156 std::string arg5 =
""
176 bool CallArmor( armor_msgs::ArmorDirective& data );
204 bool manipulationFlag =
true,
206 bool buffered_reasoner =
true
245 bool manipulationFlag =
true,
247 bool buffered_reasoner =
true
391 std::string first_spec =
"",
392 std::string second_spec =
"",
393 std::string arg1 =
"",
394 std::string arg2 =
"",
395 std::string arg3 =
"",
396 std::string arg4 =
"",
397 std::string arg5 =
"",
398 bool printRequest =
false
444 ros::ServiceClient ArmorSrv;
457 bool IsLoadedInterface =
false;
460 armor_msgs::ArmorDirectiveRes LastRes;
463 armor_msgs::ArmorDirectiveReq LastReq;
466 bool FileExist( std::string path );
#define ARMOR_DEFAULT_CLIENT
bool ConnectAndLoad(std::string path, std::string uri=ARMOR_DEFAULT_URI, bool manipulationFlag=true, std::string reasoner=ARMOR_DEFAULT_REASONER, bool buffered_reasoner=true)
connect to the server and load the ontology from file.
void PrintLastRes()
print the last response
#define ARMOR_DEFAULT_REASONER
void PrintResponse(armor_msgs::ArmorDirective &d)
print the response to the screen.
std::string GetLastErrorDescription()
last err description
armor_msgs::ArmorDirectiveReq & GetLastReq()
get a reference to the last request
#define ARMOR_DEFAULT_TIMEOUT
~ArmorTools()
class destructor (empty)
void PrintRequest(armor_msgs::ArmorDirective &d)
print a request to the screen.
void SwitchDebugMode()
toggle the debug mode
armor_msgs::ArmorDirectiveRes & GetLastRes()
get a reference to the last response
bool LoadedOntology()
check if the ontology was loaded or not
ArmorTools(std::string client=ARMOR_DEFAULT_CLIENT, std::string reference=ARMOR_DEFAULT_REFERENCE, bool dbmode=false)
Class Constructor, 3 arguments.
armor_msgs::ArmorDirective GetRequest(std::string command, std::string first_spec="", std::string second_spec="", std::string arg1="", std::string arg2="", std::string arg3="", std::string arg4="", std::string arg5="")
quick generation of an aRMOR request
bool LoadOntology(std::string path, std::string uri=ARMOR_DEFAULT_URI, bool manipulationFlag=true, std::string reasoner=ARMOR_DEFAULT_REASONER, bool buffered_reasoner=true)
load the ontology from file.
bool TestInterface()
check the status of the interface
bool UpdateOntology()
send the command REASON
bool Success()
check the 'success' flag referred to the last aRMOR call
bool Connect(float timeout=ARMOR_DEFAULT_TIMEOUT)
open a connection with the aRMOR service.
bool SendCommand(std::string command, std::string first_spec="", std::string second_spec="", std::string arg1="", std::string arg2="", std::string arg3="", std::string arg4="", std::string arg5="", bool printRequest=false)
fill in a command and send it to aRMOR
bool CallArmor(armor_msgs::ArmorDirective &data)
send a command to the aRMOR service.
A minimal C++ client for aRMOR.
bool DebugMode
debug mode enabled or not
void PrintLastReq()
print the last request
bool SaveOntology(std::string path)
save the ontology on file
#define ARMOR_DEFAULT_REFERENCE
#define ARMOR_DEFAULT_URI
int GetLastErrorCode()
err code referred to the last call